Create a Smooth Android App Navigation Experience! Navigation with Kotlin: Solve Your App Navigation Issues in One Article!
It provides a simple way to handle navigation between different fragments in an Android application.
The following example
shows how to import navigation into a project
and set up navigation.
When we complete a project
we can clearly see the entire navigation logic at a glance.
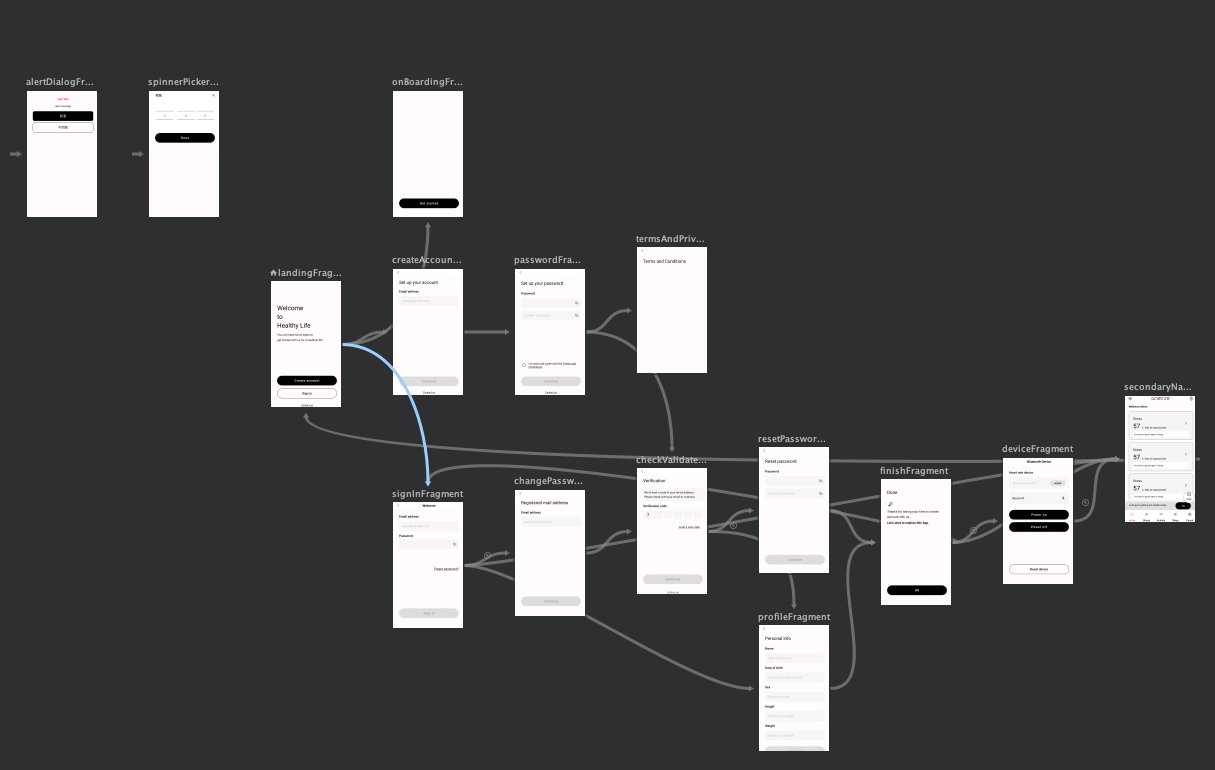
Add the relevant library to build.gradle
Note: The "Navigation" component requires Android Studio 3.3 or above
Through Android Studio
Right-click the res folder in the project directory > New > Android Resource File to add a Navigation XML.
Alternatively, if you want to add it manually, you can create a navigation folder under res and add nav_graph.xml inside it.
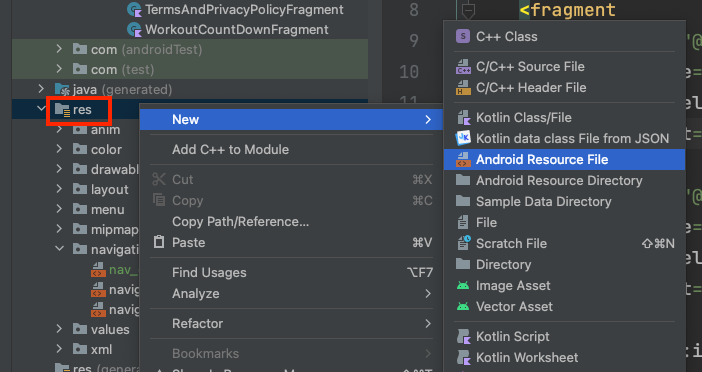
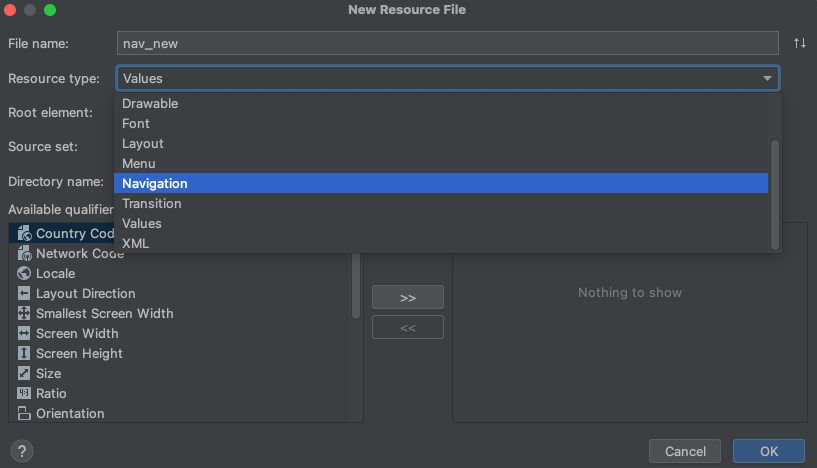
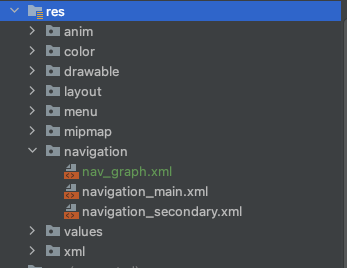
Add fragment
id = name
name = path of the fragment
label = label message or similar tag
tools:layout = layout XML resource to preview
Add startDestination
Example: app:startDestination="@id/landingFragment"
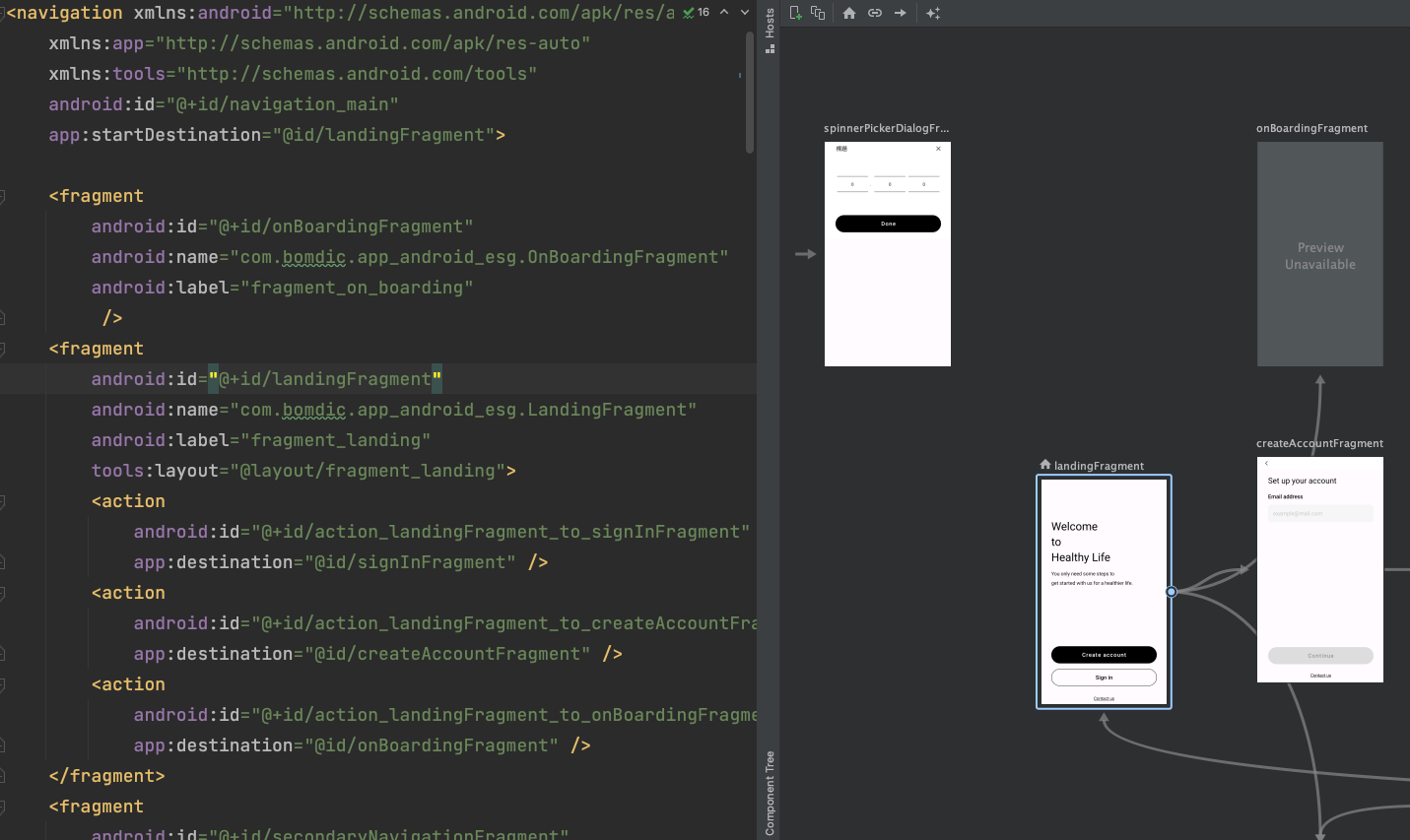
android:name="androidx.navigation.fragment.NavHostFragment"
app:navGraph="@navigation/navigation_main" (optional, adds preview in IDE)
app:defaultNavHost="true"
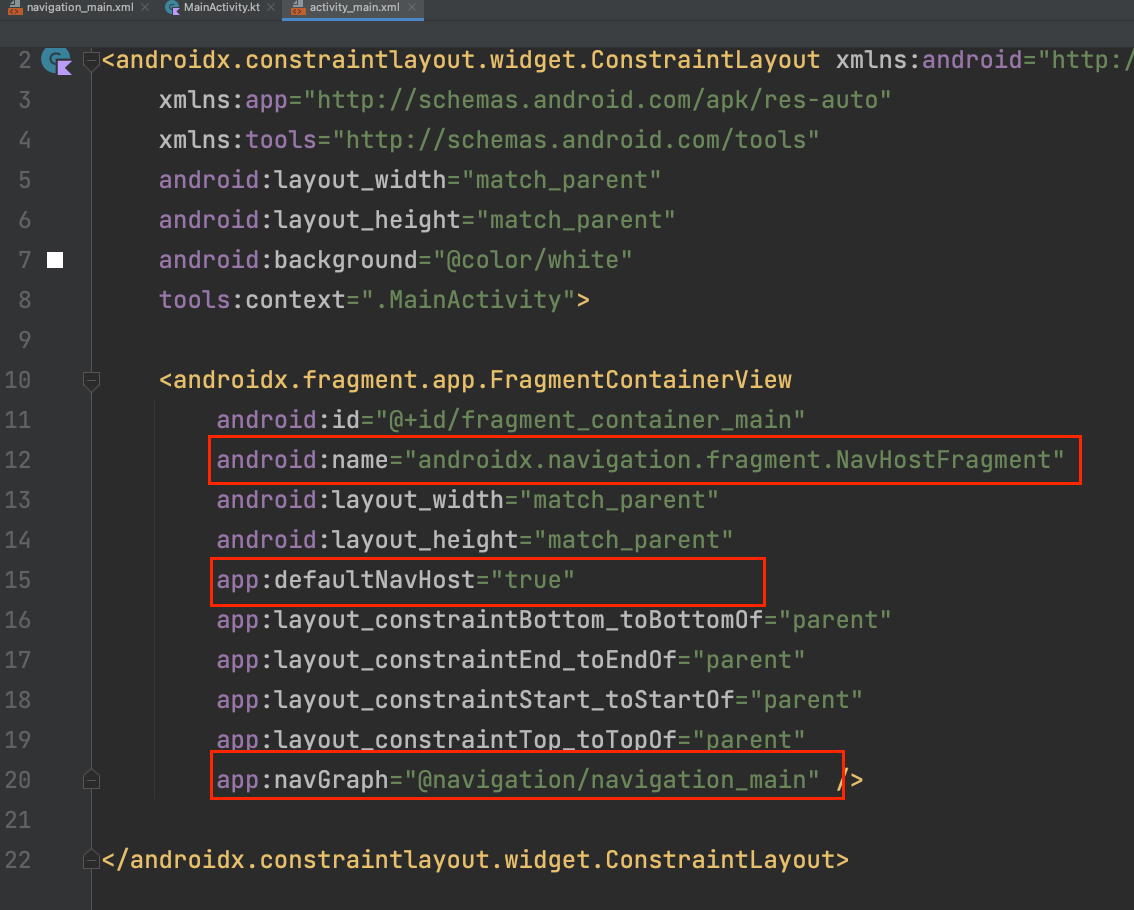
Add action within the fragment
After adding, set the destination
For example: app:destination="@id/signInFragment"
(action can also be written outside for global navigation)
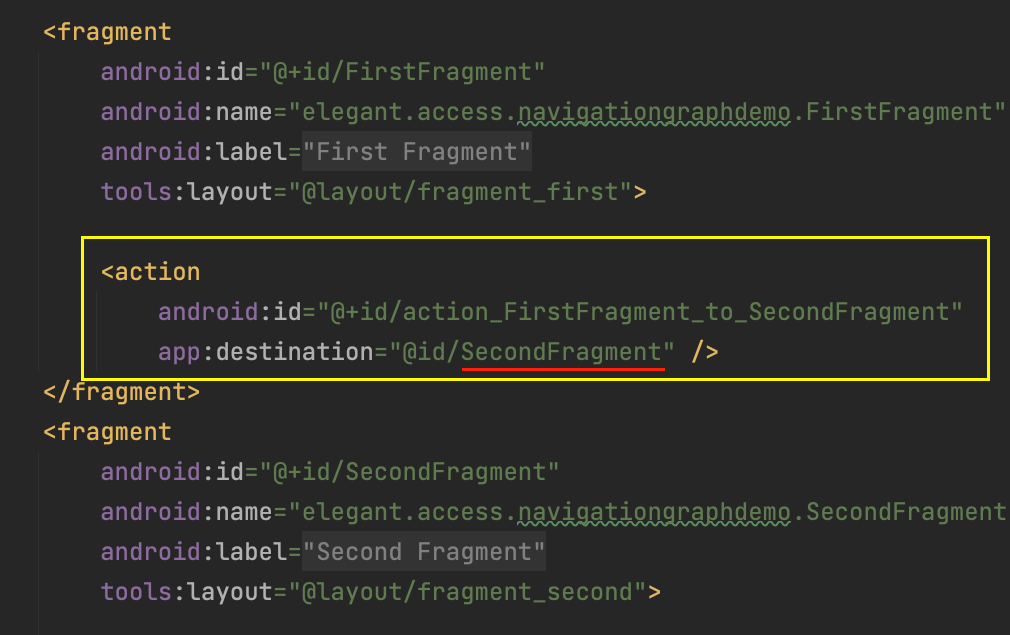
Directly add the following code where you want to navigate
findNavController().navigate(R.id.action_FirstFragment_to_SecondFragment)

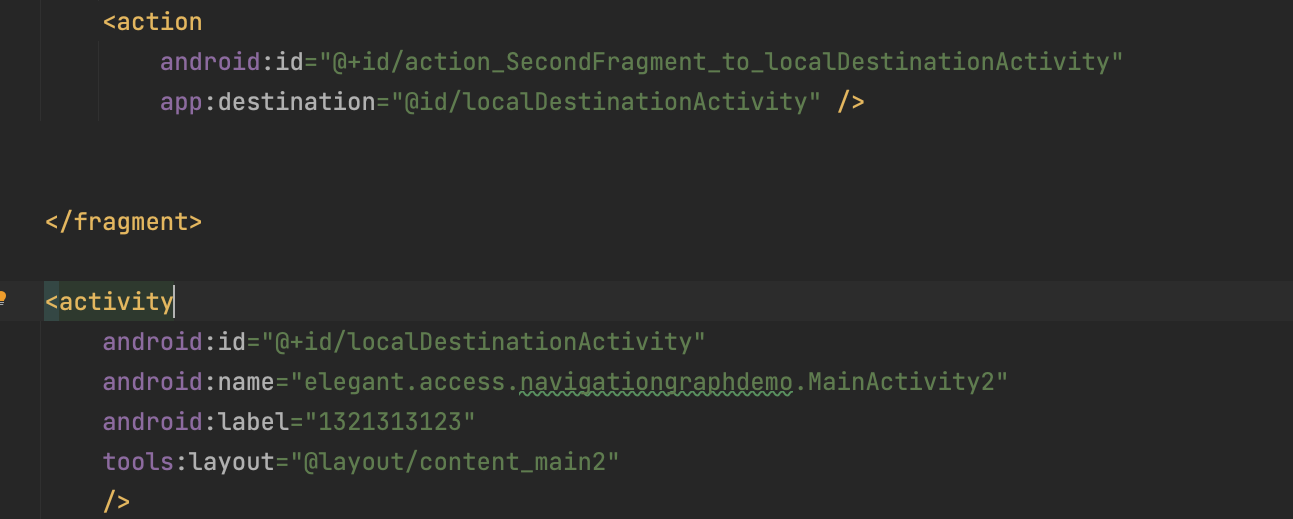
Directly add another navigation graph:
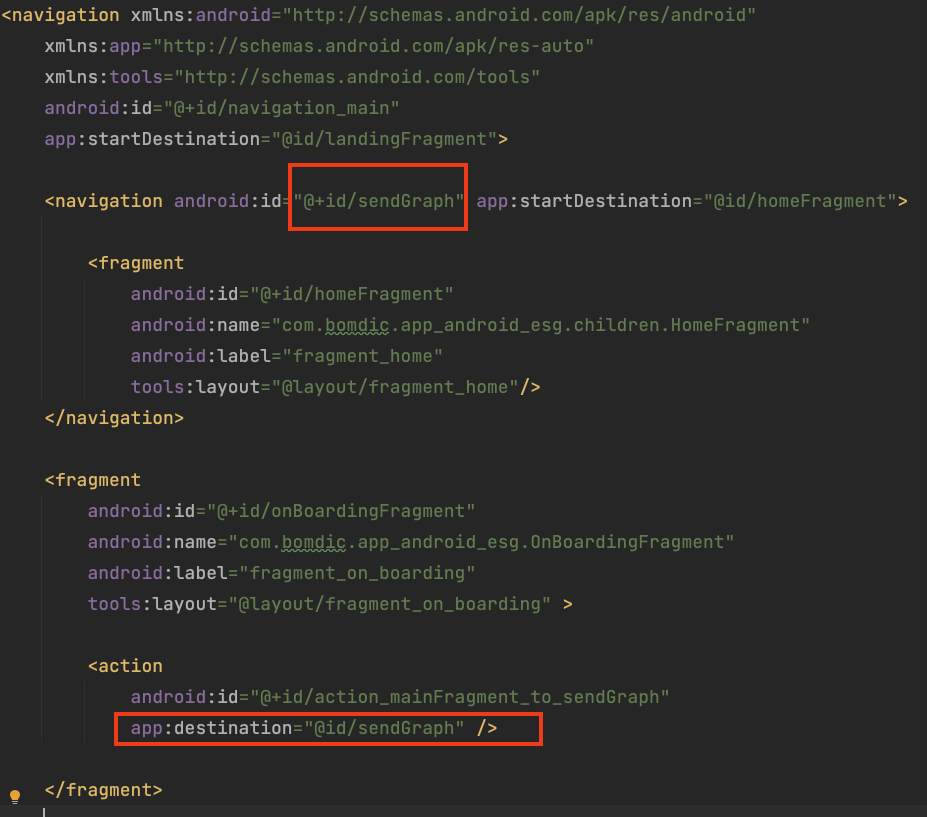
After adding a new nav graph XML
Use include to import it:
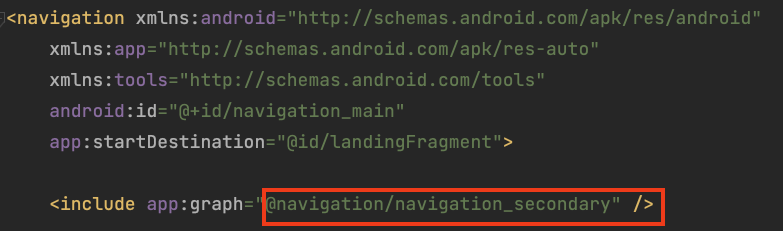
Similar to above, directly add a dialog tag in the nav graph
And import your created DialogFragment to use it
(id = name, name = fragment path, label = display message or similar tag, tools:layout = layout XML resource to display)

Similarly, directly add an argument in the nav graph
You can preset the variables to be passed
(argType = variable type, defaultValue = default value)
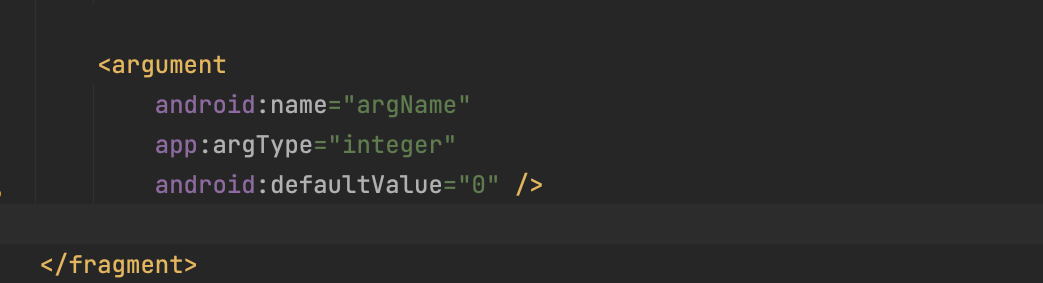
a. Without using the default value method in the above XML
You can directly pass variables using the two sets of code below
b. If default values are passed using the XML method
You can directly receive them using the code below
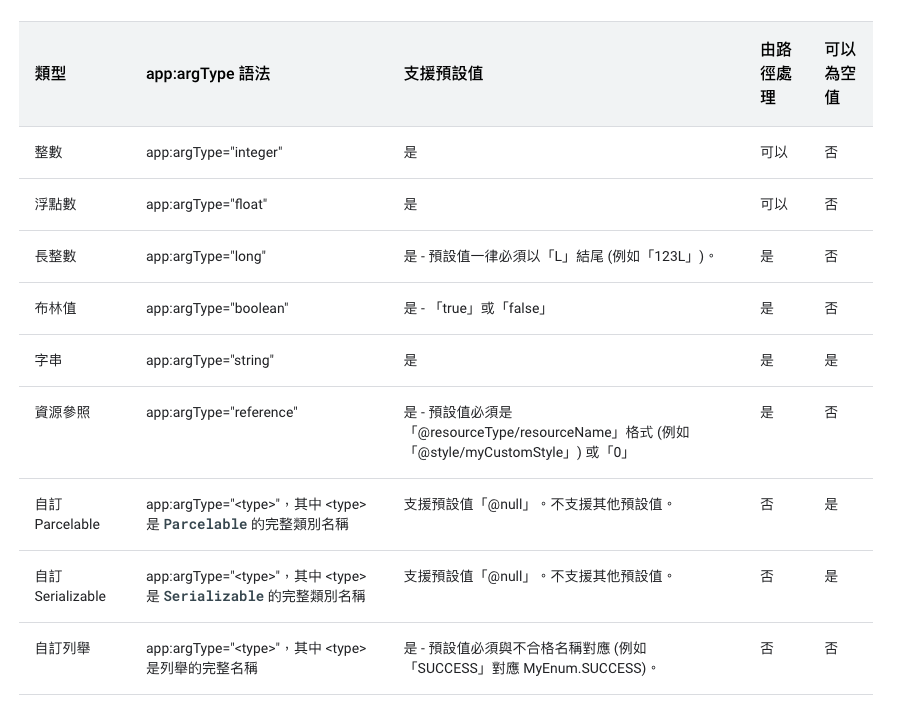
By passing enterAnim, exitAnim, popEnterAnim, and popExitAnim, you can easily set transition animations
Using the code below, you can use the built-in activity exit animations in navigation
By using the API provided by Navigation to associate related views
You can handle the issue of multiple back stack transitions
For example, setupWithNavController to associate with the bottom navigation view
setupActionBarWithNavController to associate with the action bar
This diagram illustrates the general transition logic
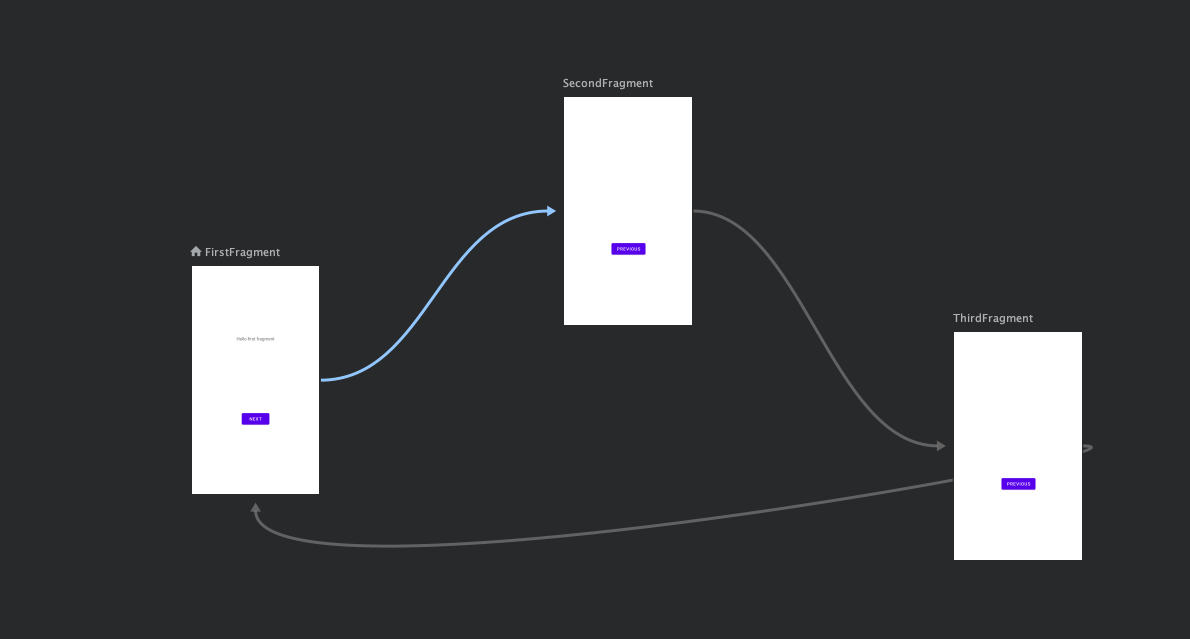
Fragments transition in order 1 -> 2 -> 3 and then back from 3 to 1
And then transition again 1 -> 2 -> 3 and back to 1
At this point, the fragments in the back stack are in the order [1,2,3,1,2,3,1]
If you want to transition back to 1 without having the destination fragment above in the stack, you can add
popUpTo and popUpToInclusive in the XML action
This will allow the next transition back to the destination fragment to have a stack instance cleared above it
Similarly, navigation also provides a corresponding builder to set some of the features mentioned above. NavOptions example:
val options = NavOptions.Builder()
.set....
.build()
Finally, pass it in when navigating
findNavController().navigate(R.id.action_FirstFragment_to_SecondFragment, null, options)
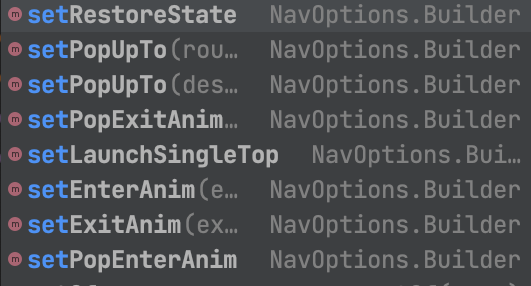